Have you ever tried using Rigol's Ultrawave software for the DG1022 function generator? Well... How can I put it mildly? It sucks.
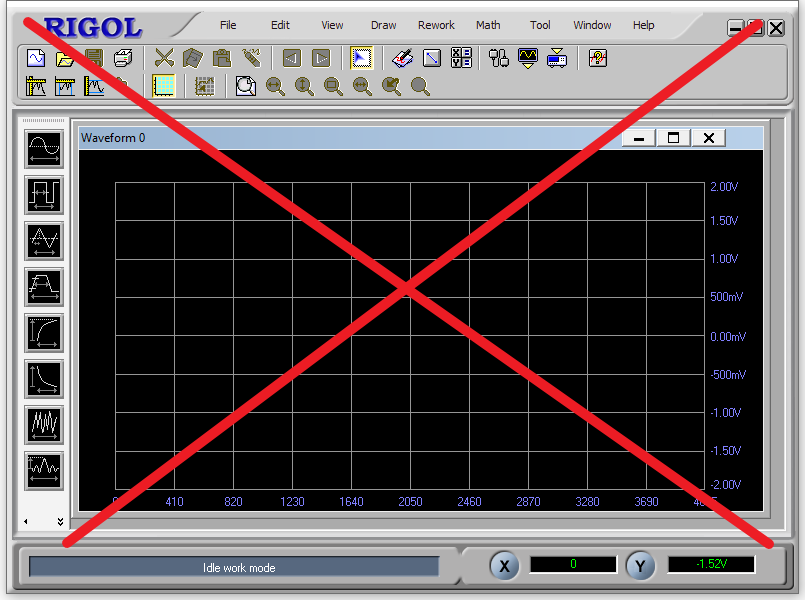
The DG1022 is a great waveform generator though. It has served me well for many years. I recently needed it to output some custom waveforms. One option would have been to write the waveforms in txt files and load them with a USB stick. Rigol's formatting is really simple. But moving the stick back and forth didn't seem like a good idea.
So I decided to write a Python script that saves waveforms to the non-volatile memory of the DG1022. Since I have no idea what kind of non-volatile memory it uses and in order not to stress it with multiple writes I also included a function that writes the waveform directly to the volatile memory and outputs it on channel 1.
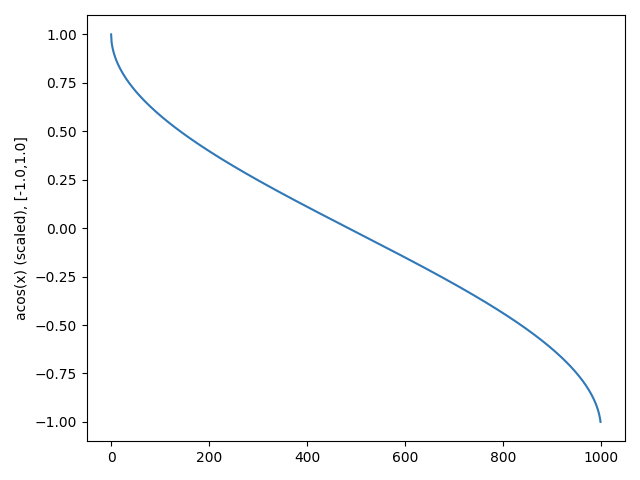